✴️ Clips Overview
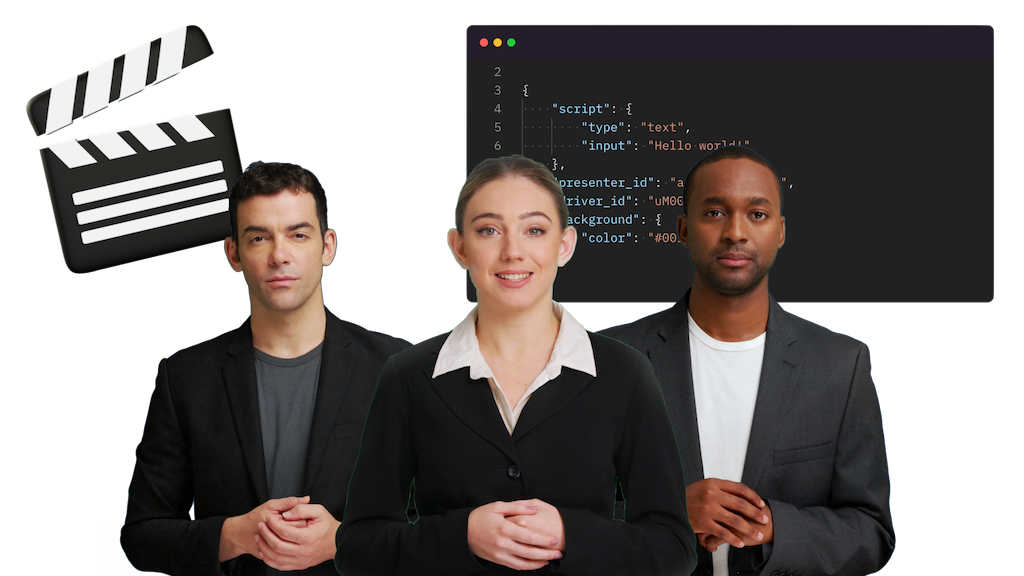
Enabling FULL-HD photorealistic avatars, medium-shot, with body and hands movements using just text or audio as input. Premium Presenters (Clips) is an easy-to-use endpoint that lets users and developers supercharge training presentations, corporate communications, sales, marketing content and more. You can also create a custom HQ Presenter in Full-HD resolution based on your own video footage.
✴️ Interface
Premium Presenter + Text or Audio file URL Output
Video URL
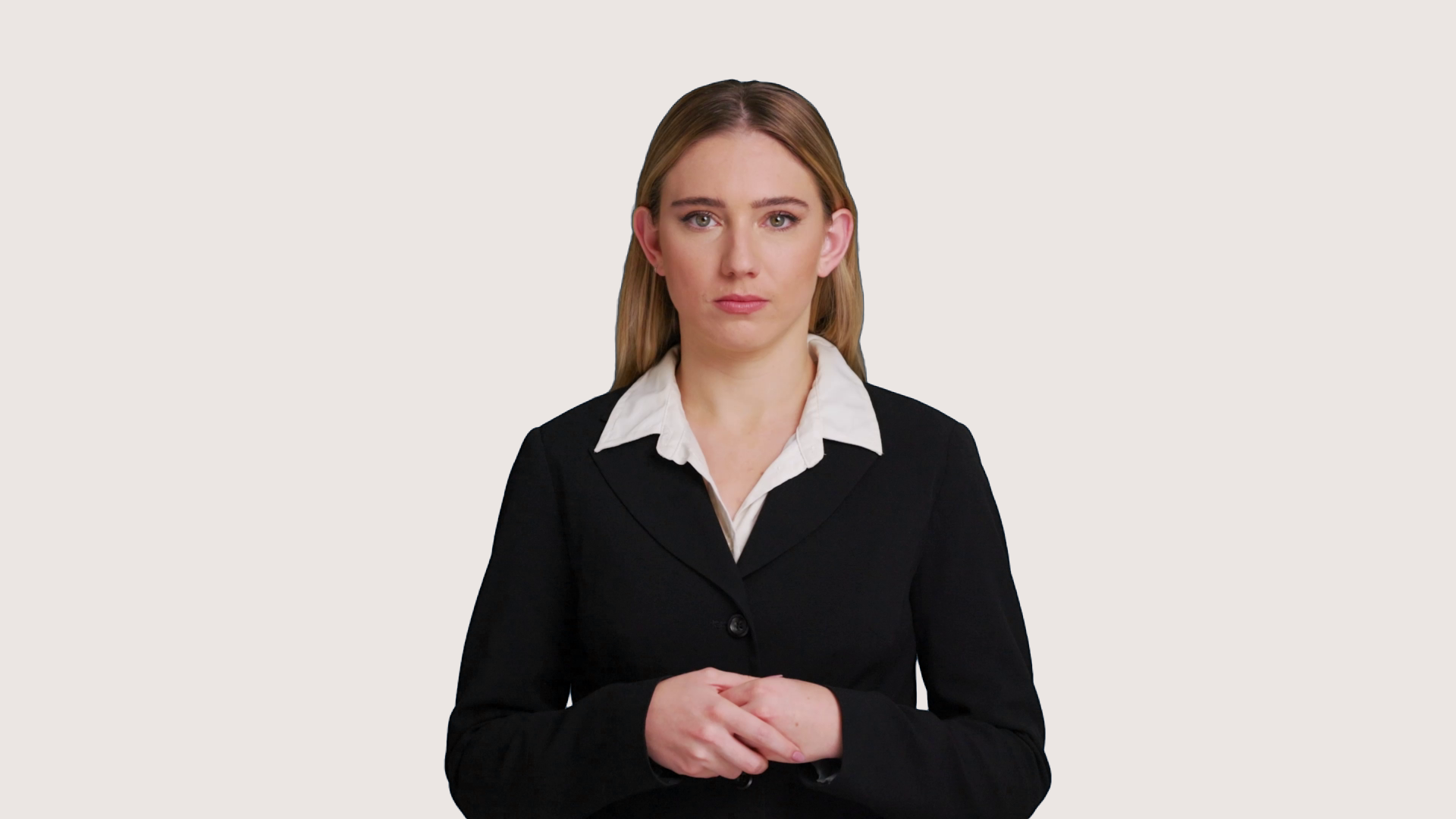
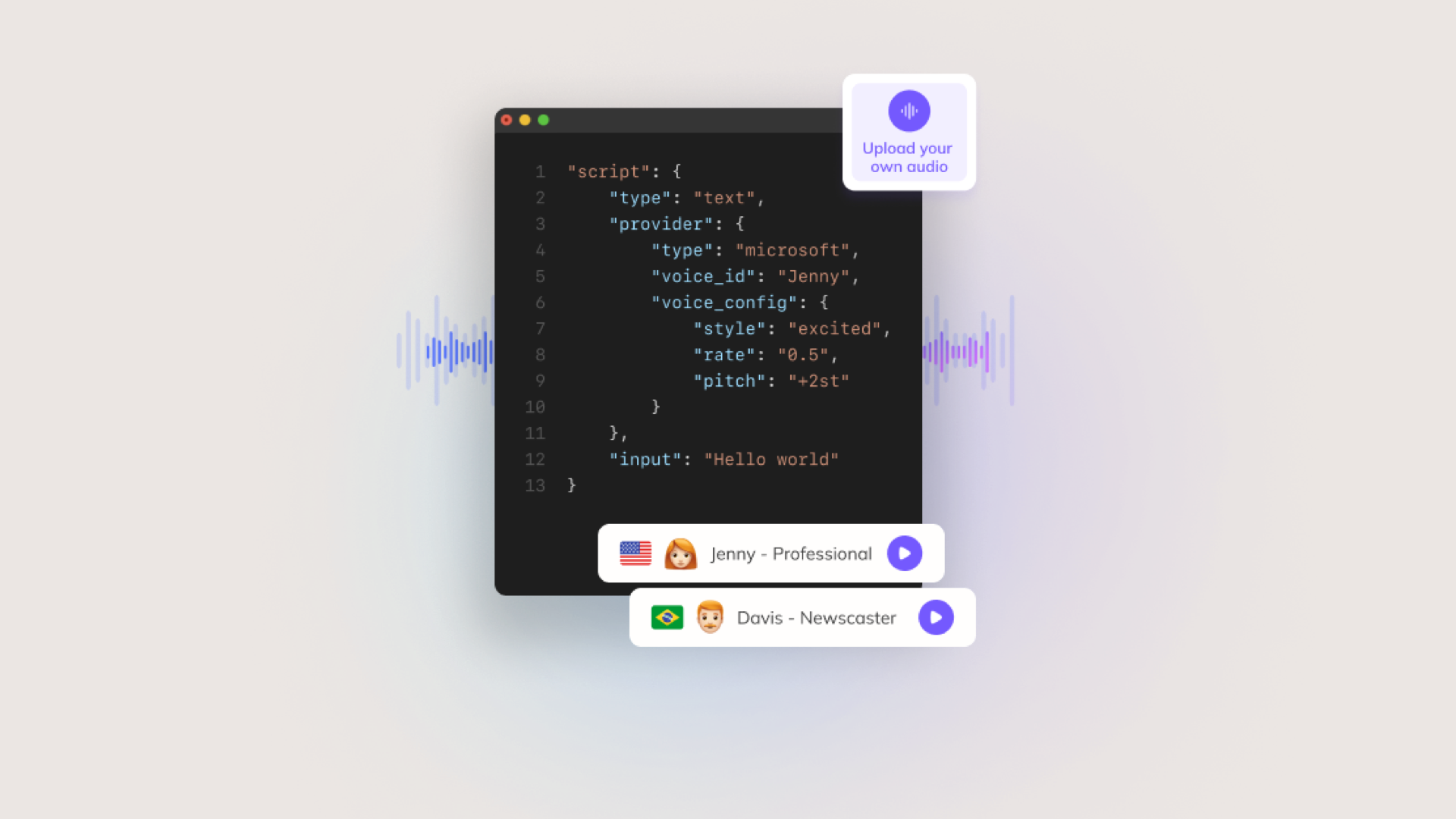
✴️ Example #1: Default Call
POST
https://api.d-id.com/clips
| Create a clip
{
"script": {
"type": "text",
"input": "Hello world!"
},
"presenter_id": "amy-Aq6OmGZnMt",
"driver_id": "Vcq0R4a8F0"
}
{
"id": "clp_auvOUSOANX95VaLCKb2Il",
"created_at": "2023-03-22T21:33:52.454Z",
"object": "clip",
"status": "created"
}
GET
https://api.d-id.com/clips/<id>
| Get a specific clip
Empty request body
See the Response tab
{
"metadata": {
"num_frames": 41,
"processing_fps": 15.429858021131948,
"resolution": [
1920,
1080
],
"size_kib": 189.255859375
},
"audio_url": "https://d-id-clips-prod.s3.us-west-2.amazonaws.com/google-oauth2%7C10396q34341765131871486/clp_auvOUSOANX95VaLCKb2Il/microsoft.wav?AWSAccessKeyId=AKIA5SDFDSFIJ7CPKJNP&Expires=1679607232&Signature=%2Fo97jLpizsFfunCJKjEseb8OsPE%3D",
"created_at": "2023-03-22T21:33:52.454Z",
"config": {
"logo": {
"url": "ai",
"position": [
0,
0
]
},
"result_format": ".mp4"
},
"source_url": "https://clips-presenters.d-id.com/amy/jcwCkr1grs/uM00QMwJ9x/thumbnail.png",
"created_by": "google-oauth2|12345678",
"status": "done",
"driver_id": "uM00QMwJ9x",
"modified_at": "2023-03-22T21:34:01.025Z",
"completed_at": "2023-03-22T21:34:00.924",
"presenter_id": "amy-jcwCkr1grs",
"result_url": "https://d-id-clips-prod.s3.us-west-2.amazonaws.com/google-oauth2%7C1039658317634543586/clp_auvOUSOADFGDFKb2Il/amy-jcwCkr1grs.mp4?AWSAccessKeyId=AKIA5CUMPJBIJ7CPKJNP&Expires=1679607241&Signature=UjgamgTtNfSNL2IUiOOMSMBOI0U%3D",
"id": "clp_auvOUSOANX95VaLCKb2Il",
"duration": 2,
"started_at": "2023-03-22T21:33:52.978",
"owner_id": "google-oauth2|12345678"
}
The output video is located in the result_url
field.
Note
The output video is ready only when
"status": "done"
status
field lifecycle:
"status": "created" | When posting a new clips request |
"status": "started" | When starting the video processing |
"status": "done" | When the video is ready |
Default presenters list:
actor_id | presenter_id | driver_id |
---|---|---|
amy | amy-Aq6OmGZnMt | Vcq0R4a8F0 |
jack | jack-Pt27VkP3hW | fbQicImV2J |
matt | matt-g7muIj5CiD | w8bWNn8GSl |
Full Presenters list
In order to get the most updated presenters list, you should make a call to
/clips/presenters
endpointGet Presenters endpoint also contains video examples of the presenters
GET
https://api.d-id.com/clips/presenters?limit=1000
| Get presenters list
Empty request body
See the Response tab
{
"presenters": [
{
"presenter_id": "rian-pbMoTzs7an",
"created_at": "2023-08-20T12:53:44.254Z",
"thumbnail_url": "https://clips-presenters.d-id.com/rian/pbMoTzs7an/vmcS2q23Kk/thumbnail.png",
"preview_url": "https://clips-presenters.d-id.com/rian/pbMoTzs7an/vmcS2q23Kk/preview.mp4",
"driver_id": "vmcS2q23Kk",
"image_url": "https://clips-presenters.d-id.com/rian/pbMoTzs7an/vmcS2q23Kk/image.png",
"gender": "male",
"model_url": "s3://d-id-clips-drivers-prod/rian/pbMoTzs7an/generator.pt",
"modified_at": "2023-09-27T11:06:44.603Z",
"owner_id": ""
},
{
"presenter_id": "rian-lZC6MmWfC1",
"created_at": "2023-08-20T12:53:52.505Z",
"thumbnail_url": "https://clips-presenters.d-id.com/rian/lZC6MmWfC1/mXra4jY38i/thumbnail.png",
"preview_url": "https://clips-presenters.d-id.com/rian/lZC6MmWfC1/mXra4jY38i/preview.mp4",
"driver_id": "mXra4jY38i",
"image_url": "https://clips-presenters.d-id.com/rian/lZC6MmWfC1/mXra4jY38i/image.png",
"gender": "male",
"model_url": "s3://d-id-clips-drivers-prod/rian/lZC6MmWfC1/generator.pt",
"modified_at": "2023-09-27T11:06:42.910Z",
"owner_id": ""
},
{
"presenter_id": "rian-bYZilf8Qr9",
"created_at": "2023-08-20T12:54:07.568Z",
"thumbnail_url": "https://clips-presenters.d-id.com/rian/bYZilf8Qr9/UoaR8NoiQo/thumbnail.png",
"preview_url": "https://clips-presenters.d-id.com/rian/bYZilf8Qr9/UoaR8NoiQo/preview.mp4",
"driver_id": "UoaR8NoiQo",
"image_url": "https://clips-presenters.d-id.com/rian/bYZilf8Qr9/UoaR8NoiQo/image.png",
"gender": "male",
"model_url": "s3://d-id-clips-drivers-prod/rian/bYZilf8Qr9/generator.pt",
"modified_at": "2023-09-27T11:06:41.412Z",
"owner_id": ""
},
{
"presenter_id": "lana-uXbrIxQFjr",
"created_at": "2023-08-21T14:08:49.519Z",
"thumbnail_url": "https://clips-presenters.d-id.com/lana/uXbrIxQFjr/kzlKYBZ2wc/thumbnail.png",
"preview_url": "https://clips-presenters.d-id.com/lana/uXbrIxQFjr/kzlKYBZ2wc/preview.mp4",
"driver_id": "kzlKYBZ2wc",
"image_url": "https://clips-presenters.d-id.com/lana/uXbrIxQFjr/kzlKYBZ2wc/image.png",
"gender": "female",
"model_url": "s3://d-id-clips-drivers-prod/lana/uXbrIxQFjr/generator.pt",
"modified_at": "2023-09-27T11:07:28.531Z",
"owner_id": ""
},
And many more!
Drivers list
In order to get the most updated drivers list per presenter, you should make a call to
/clips/presenters/<id>/drivers
endpoint
GET
https://api.d-id.com/clips/presenters/{id}/drivers
| Get drivers list of an actor
Empty request body
See the Response tab
{
"clips_drivers": [
{
"presenter_id": "amy-jcwCkr1grs",
"driver_id": "uM00QMwJ9x",
"driver_type": "scene",
"gender": "female",
"created_at": "2022-09-06T09:55:02.599Z",
"modified_at": "2022-09-06T09:57:38.704Z",
"driver_image_url": "https://clips-presenters.d-id.com/amy/jcwCkr1grs/uM00QMwJ9x/image.png",
"thumbnail_url": "https://clips-presenters.d-id.com/amy/jcwCkr1grs/uM00QMwJ9x/thumbnail.png",
"video_url": "https://clips-presenters.d-id.com/amy/jcwCkr1grs/uM00QMwJ9x/video.mp4",
"preview_url": "https://clips-presenters.d-id.com/amy/jcwCkr1grs/uM00QMwJ9x/preview.mp4"
}
]
}
✴️ Example #2: Webhooks
Simply create an endpoint on your side and add it in the webhook
field.
Then the webhook endpoint will be triggered with the same response body once the video is ready.
POST
https://api.d-id.com/clips
| Create a clip
{
"script": {
"type": "text",
"input": "Hello world!"
},
"presenter_id": "amy-Aq6OmGZnMt",
"driver_id": "Vcq0R4a8F0",
"webhook": "https://myhost.com/webhook"
}
{
"id": "clp_auvOUSOANX95VaLCKb2Il",
"created_at": "2023-03-22T21:33:52.454Z",
"object": "clip",
"status": "created"
}
{
"metadata": {
"num_frames": 41,
"processing_fps": 15.429858021131948,
"resolution": [
1920,
1080
],
"size_kib": 189.255859375
},
"audio_url": "https://d-id-clips-prod.s3.us-west-2.amazonaws.com/google-oauth2%7C10396q34341765131871486/clp_auvOUSOANX95VaLCKb2Il/microsoft.wav?AWSAccessKeyId=AKIA5SDFDSFIJ7CPKJNP&Expires=1679607232&Signature=%2Fo97jLpizsFfunCJKjEseb8OsPE%3D",
"created_at": "2023-03-22T21:33:52.454Z",
"config": {
"logo": {
"url": "ai",
"position": [
0,
0
]
},
"result_format": ".mp4"
},
"source_url": "https://clips-presenters.d-id.com/amy/jcwCkr1grs/uM00QMwJ9x/thumbnail.png",
"created_by": "google-oauth2|12345678",
"status": "done",
"driver_id": "uM00QMwJ9x",
"modified_at": "2023-03-22T21:34:01.025Z",
"completed_at": "2023-03-22T21:34:00.924",
"presenter_id": "amy-jcwCkr1grs",
"result_url": "https://d-id-clips-prod.s3.us-west-2.amazonaws.com/google-oauth2%7C1039658317634543586/clp_auvOUSOADFGDFKb2Il/amy-jcwCkr1grs.mp4?AWSAccessKeyId=AKIA5CUMPJBIJ7CPKJNP&Expires=1679607241&Signature=UjgamgTtNfSNL2IUiOOMSMBOI0U%3D",
"id": "clp_auvOUSOANX95VaLCKb2Il",
"duration": 2,
"started_at": "2023-03-22T21:33:52.978",
"owner_id": "google-oauth2|12345678"
}
✴️ Example #3: Text to Speech
Choose different voices, languages, and styles. See the supported Text-to-Speech providers' voices list
POST
https://api.d-id.com/clips
| Create a clip
{
"script": {
"type": "text",
"input": "Hello world!",
"provider": {
"type": "microsoft",
"voice_id": "en-US-JennyNeural",
"voice_config": {
"style": "Cheerful"
}
}
},
"presenter_id": "amy-Aq6OmGZnMt",
"driver_id": "Vcq0R4a8F0"
}
{
"id": "clp_auvOUSOANX95VaLCKb2Il",
"created_at": "2023-03-22T21:33:52.454Z",
"object": "clip",
"status": "created"
}
✴️ Example #4: Audio script
Using an audio file instead of a text
POST
https://api.d-id.com/clips
| Create a clip
{
"script": {
"type": "audio",
"audio_url": "https://path.to/audio.mp3"
},
"presenter_id": "amy-Aq6OmGZnMt",
"driver_id": "Vcq0R4a8F0"
}
{
"id": "clp_auvOUSOANX95VaLCKb2Il",
"created_at": "2023-03-22T21:33:52.454Z",
"object": "clip",
"status": "created"
}
✴️ Example #5: Background
Change the background color. Use HEX color format.
POST
https://api.d-id.com/clips
| Create a clip
{
"script": {
"type": "audio",
"audio_url": "https://path.to/audio.mp3"
},
"presenter_id": "amy-Aq6OmGZnMt",
"driver_id": "Vcq0R4a8F0",
"background": {
"color": "#aa22cc"
}
}
{
"id": "clp_auvOUSOANX95VaLCKb2Il",
"created_at": "2023-03-22T21:33:52.454Z",
"object": "clip",
"status": "created"
}
Transparent background. Available only in webm
format.
POST
https://api.d-id.com/clips
| Create a clip
{
"script": {
"type": "audio",
"audio_url": "https://path.to/audio.mp3"
},
"presenter_id": "amy-Aq6OmGZnMt",
"driver_id": "Vcq0R4a8F0",
"config": {
"result_format": "webm"
},
"background": {
"color": false
}
}
{
"id": "clp_auvOUSOANX95VaLCKb2Il",
"created_at": "2023-03-22T21:33:52.454Z",
"object": "clip",
"status": "created"
}
✴️ Video Tutorial
Live Coding Session
✴️ Support
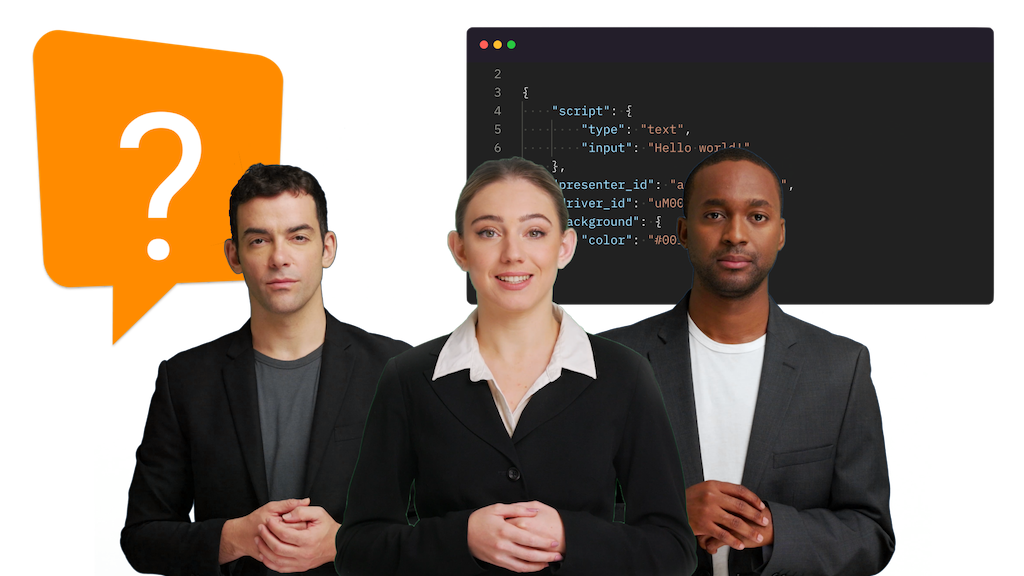
Have any questions? We are here to help! Please leave your question in the Discussions section and we will be happy to answer shortly.
Ask a question